Choosing the Right Tech Stack: Neon, Node.js, React & Clerk for an MVP
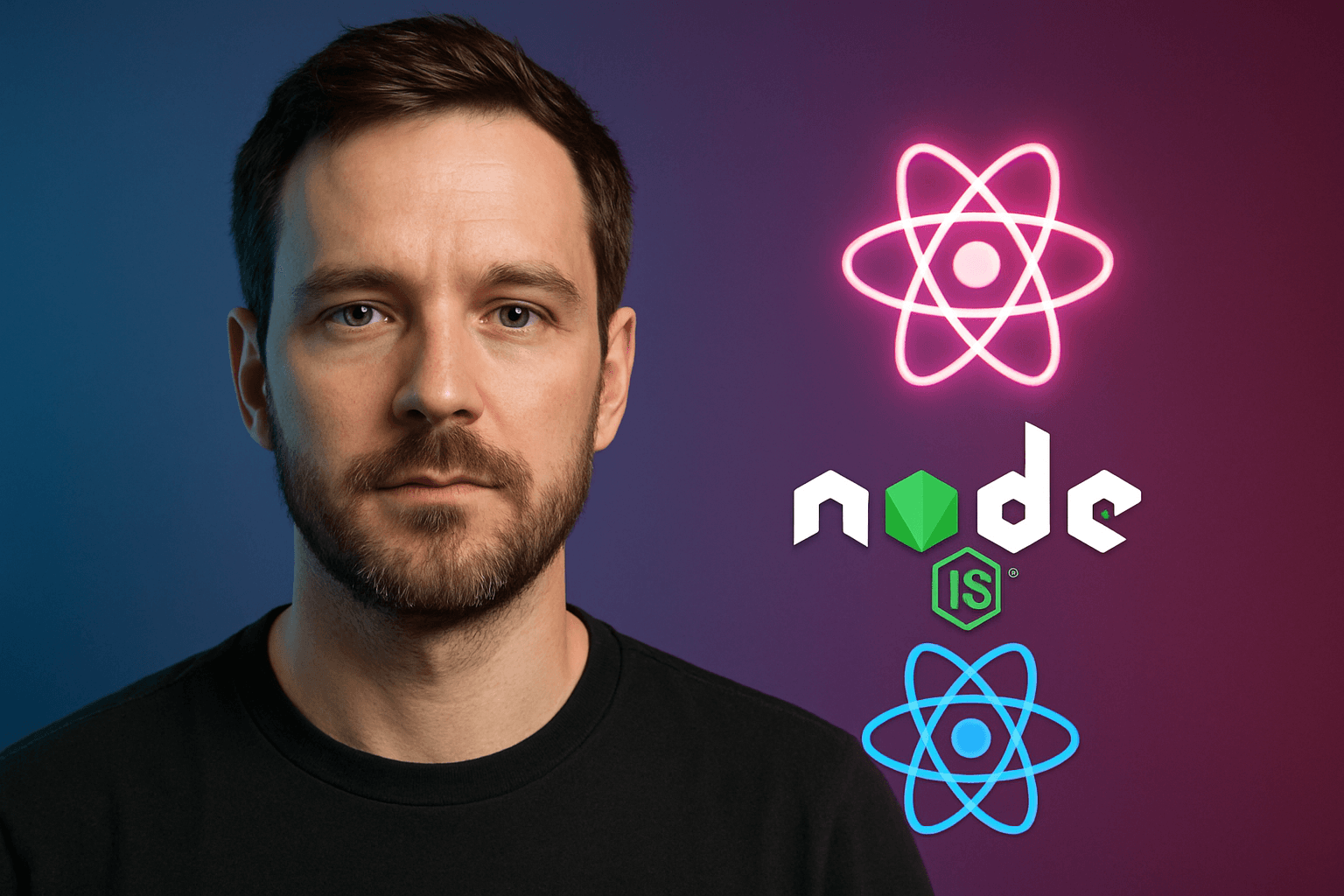
Introduction: Tech Choices That Accelerate MVPs
Choosing the right technology stack is one of the most critical decisions when building a new web application, especially a Minimum Viable Product (MVP) or an internal tool. The wrong choices can lead to slow development, scalability issues, and maintenance headaches down the road. The right choices, however, can significantly accelerate development, improve reliability, and provide a solid foundation for future growth.
In my recent project migrating an [Link to Excel Migration Post]SEO workflow from Excel to a custom web app[/Link], I needed a stack that balanced speed, flexibility, scalability, and developer experience. This wasn't about building a massive enterprise system; it was about delivering core value quickly and efficiently.
This article breaks down the specific technologies I selected – Neon (Serverless PostgreSQL), Node.js, React with TypeScript, and Clerk – explaining the rationale behind each choice, the specific benefits they offered, and how they integrated seamlessly to power the MVP.
The Core Challenge: Build Fast, Stay Flexible, Be Secure
Before diving into individual technologies, let's recap the project's core requirements, which heavily influenced the tech stack decisions:
- Rapid Development: The primary goal was to replace a time-consuming manual process quickly. The stack needed to minimize boilerplate and allow for fast iteration.
- Scalability (On Demand): While starting small, the database and backend needed to handle potentially growing amounts of SEO data (rankings, backlinks over time) without requiring manual server provisioning or complex scaling configurations.
- Asynchronous Operations: The application needed to reliably fetch data from external SEO APIs on a schedule (e.g., daily or weekly).
- Dynamic & Interactive UI: A responsive dashboard with charts, tables, and filtering was essential for replacing the spreadsheet's analytical capabilities.
- Secure Authentication: As multiple users (consultant and clients) needed access, robust and easy-to-implement authentication was mandatory.
Database Deep Dive: Why Neon for Serverless PostgreSQL?
Data storage is foundational. While NoSQL options exist, the relational nature of SEO data (projects, keywords, rankings, backlinks, users, relationships between them) made PostgreSQL a strong candidate. But traditional managed PostgreSQL can involve provisioning, scaling concerns, and potentially higher costs for an MVP. Enter Neon.
What is Neon?
Neon is a fully-managed, serverless PostgreSQL platform. It separates storage and compute, allowing for features like instant database branching, scaling to zero when inactive, and usage-based pricing.
Key Advantages of Neon for this Project:
- Truly Serverless: No need to choose instance sizes or worry about scaling compute. It handles load automatically and can scale down to zero, making the free/hobby tier incredibly cost-effective for MVPs or internal tools.
- Rapid Setup: Creating a new PostgreSQL database literally takes seconds through their dashboard. Connection pooling is often handled automatically or easily configured.
- Database Branching: This was a killer feature for development. Create instant, isolated branches of your production database for testing new features or schema changes without affecting live data. Merge changes back if successful. Incredibly powerful for safe iteration.
- Developer Experience (DX): Modern integrations, clear documentation, and compatibility with standard PostgreSQL clients made it easy to connect from Node.js. Support for edge functions is a bonus for certain architectures.
- PostgreSQL Power: You get the full power of PostgreSQL (SQL queries, JSONB support, extensions) without the operational overhead.
Potential Tradeoffs to Consider:
- Cold Starts: In the free/lower tiers, an inactive database might take a few seconds to "wake up" on the first connection after a period of inactivity. This was acceptable for this internal tool but might be a concern for high-traffic, latency-sensitive public applications.
- Maturity & Feature Set: While robust, it might lack some highly specific enterprise features or integrations found in platforms like AWS RDS or Google Cloud SQL, though this gap is constantly closing.
Verdict: For an MVP needing the structure of SQL with the flexibility and cost-effectiveness of serverless, Neon was an outstanding choice, significantly simplifying database management.
Frontend Framework: React + TypeScript – The Reliable Standard
For the user-facing dashboard, React paired with TypeScript was the natural fit.
Why React & TypeScript?
- Component-Based UI: React's model makes building complex, reusable UI elements like data tables, charts, and filter controls manageable.
- Rich Ecosystem: Access to a vast library of components, charting libraries (like Recharts or Nivo), and state management solutions (though for this MVP, basic
useState
/useContext
or a lightweight library like Zustand/Jotai was sufficient). SWR or React Query are excellent for data fetching and caching. - TypeScript for Safety: In any application involving data manipulation and API calls, TypeScript's static typing is invaluable. It catches potential errors during development (e.g., passing the wrong data type, accessing non-existent properties), making the codebase more robust and easier to refactor.
- Developer Pool: React remains one of the most popular frontend libraries, making it easier to find resources or collaborate if needed.
Frontend Features Implemented:
- Interactive Dashboard: Displaying key SEO metrics, ranking trends, and backlink summaries. [Placeholder: Screenshot of dashboard UI]
- Data Tables: Sortable and filterable tables for detailed keyword/backlink views.
- Filtering Controls: Dropdowns and input fields to filter data by project, keyword group, date range, etc.
- Basic Charting: Visualizing trends over time.
- Responsive Design: Ensuring usability on different screen sizes using Tailwind CSS.
Verdict: React + TypeScript provided the power and safety needed to build a functional and maintainable frontend quickly, leveraging the large ecosystem without unnecessary complexity.
Backend Engine: Node.js – Efficient & Asynchronous
The backend acts as the crucial intermediary, handling business logic, data fetching, and API interactions.
Why Node.js?
- Asynchronous Nature: Node.js excels at I/O-bound tasks, making it perfect for fetching data from multiple external SEO APIs concurrently without blocking.
- JavaScript Ecosystem: Using JavaScript/TypeScript on both frontend and backend can streamline development ("full-stack JavaScript"). Access to npm provides countless libraries for tasks like API clients, scheduling (e.g.,
node-cron
), and data manipulation. - Lightweight & Fast Prototyping: Frameworks like Express allow for rapid API development. For serverless deployments (e.g., Vercel Functions, AWS Lambda), Node.js is a well-supported and efficient choice.
- Easy Integration: Simple to connect to Neon PostgreSQL (using libraries like
pg
or an ORM like Prisma) and Clerk.
Backend Responsibilities:
- API Endpoints: Providing RESTful endpoints for the React frontend to fetch processed SEO data (e.g.,
/api/projects/:id/rankings
). - Scheduled Tasks: Running background jobs (e.g., using
node-cron
or a serverless function trigger) to periodically fetch fresh data from SEO APIs and update the Neon database. - Data Processing/Aggregation: Performing necessary calculations or data transformations before sending data to the frontend.
- Authentication Middleware: Integrating with Clerk to protect API endpoints.
Verdict: Node.js offered the right balance of performance, ecosystem support, and development speed needed for the backend logic and API integrations.
Authentication Layer: Clerk – Secure & Simple
As covered previously, handling authentication securely is critical but time-consuming to build from scratch.
Why Clerk?
- Pre-Built UI & Logic: Saved dozens of hours by providing ready-to-use components for sign-in, sign-up, user profiles, and session management.
- Security Focused: Offloads the complexities of password hashing, session handling, and vulnerability patching to a specialized service.
- Easy Integration: React hooks (
useUser
,useAuth
) and Node.js middleware (ClerkExpressRequireAuth
) made connecting the frontend and backend seamless. - Feature Rich: Supports social logins, multi-factor authentication, and organization management out-of-the-box if needed later.
Verdict: Clerk was instrumental in launching quickly with robust, secure authentication, allowing focus to remain on the core SEO features. [Link to your Clerk Article]
Synergy: How the Stack Worked Together
The beauty of this stack lies in how well the components complement each other:
- User Interaction: The user interacts with the React + TypeScript frontend.
- Authentication: Clerk handles login/signup via its UI components. Requests from authenticated users include a JWT.
- API Request: The React app makes requests to the Node.js backend API.
- Auth Verification: Clerk's middleware on the Node.js backend verifies the JWT, making user information available (
req.auth
). - Data Retrieval/Logic: The Node.js backend queries the Neon PostgreSQL database for user-specific data, potentially fetching fresh data from external APIs if needed.
- Response: The Node.js backend sends the processed data back to the React frontend for display.
- Background Tasks: Scheduled Node.js tasks independently fetch data from SEO APIs and update the Neon database.
[Placeholder: Simple architecture diagram showing React -> Node.js -> Neon with Clerk handling auth]
Surprising Wins and Key Takeaways
- Neon's Branching: This feature cannot be overstated for development velocity and safety. It fundamentally changes how you can test database-related changes.
- Clerk's DX: The ease of dropping in auth components felt almost too simple compared to traditional methods.
- PostgreSQL + JSONB Flexibility: Storing less structured metadata (like API responses or configuration details) within JSONB columns in PostgreSQL offered NoSQL-like flexibility alongside relational integrity.
This stack proved to be more than just functional; it was genuinely enjoyable to work with, promoting rapid iteration and confidence in the resulting application.
Final Thoughts: A Solid Foundation for MVPs
For developers building internal tools, SaaS MVPs, or custom dashboards – especially those dealing with structured data and requiring user authentication – this combination of Neon, Node.js, React/TypeScript, and Clerk offers a compelling blend of:
- Development Speed: Minimize boilerplate and leverage pre-built solutions.
- Scalability: Start small with serverless components that can grow.
- Modern Developer Experience: Leverage strong typing, great tooling, and active communities.
- Cost-Effectiveness: Generous free tiers (Neon, Clerk) make it ideal for bootstrapping.
While every project has unique needs, this stack provides a powerful, versatile, and efficient foundation for turning ideas – even those trapped in spreadsheets – into functional web applications.
What tech stack challenges are you facing with your current project? Let me know in the comments!
Related Tags:
Tech Stack, MVP Development, PostgreSQL, Serverless Database, Neon Database, React, Node.js, TypeScript, Clerk Auth, Web Development, Software Architecture, Case Study