Clerk React Auth Tutorial: Secure Setup Guide (2025)
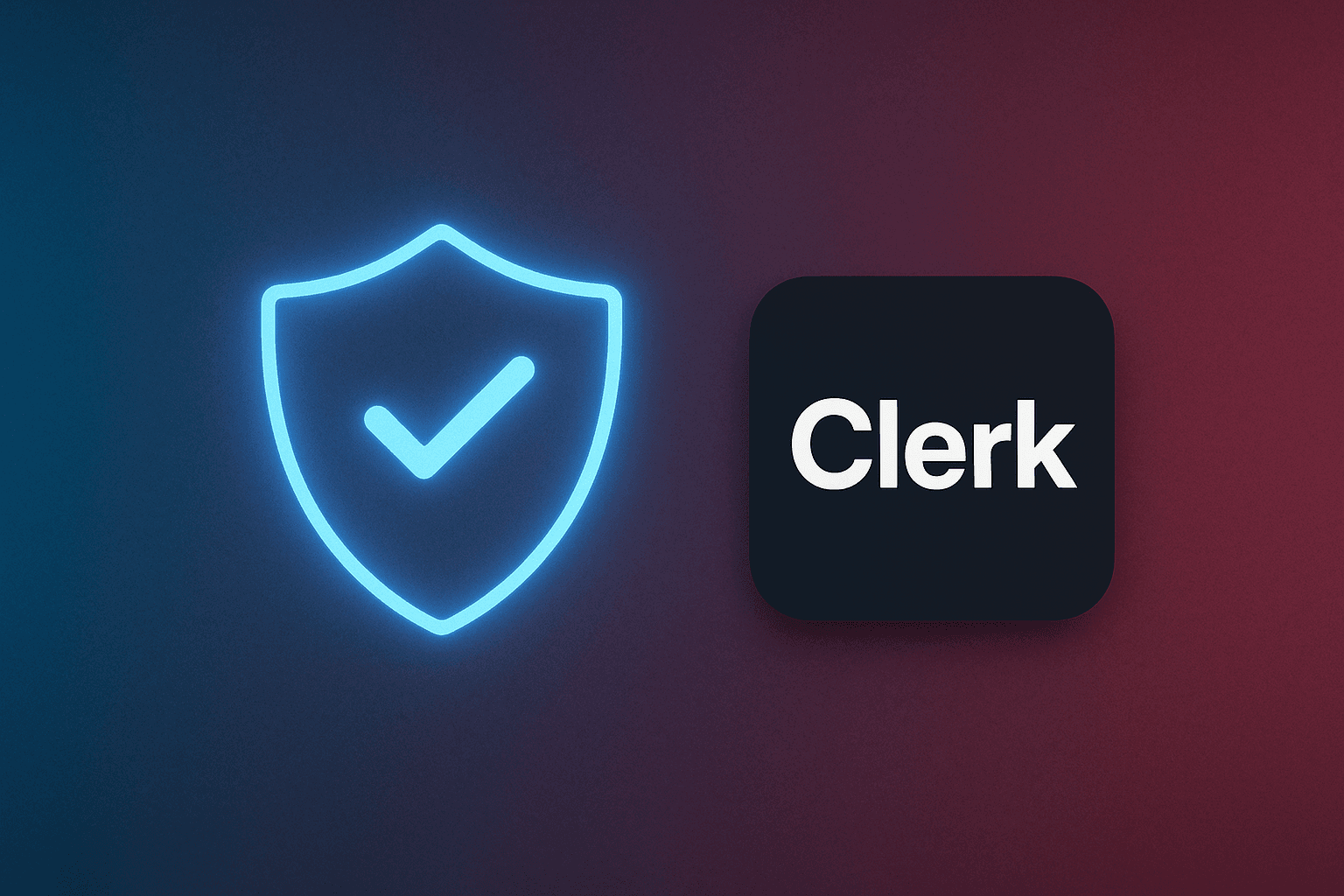
Introduction: The Auth Bottleneck
When building a web app — whether it's a full-blown SaaS or a focused internal tool — user authentication is almost always a non-negotiable requirement. But let's be honest: setting up auth from scratch is a notorious time sink, riddled with potential security pitfalls.
Handling sign-up forms, password hashing, email verifications, secure session management, social logins... it's a lot. Especially if you're a solo developer or a small team trying to ship an MVP, building robust auth can feel like a major distraction from your core product. Security mistakes are easy to make and can be incredibly costly.
In this post, I'll walk you through why I chose Clerk for secure authentication in a recent [Link to previous SEO App post]SEO automation project[/Link], how it stacks up against popular alternatives, and provide a step-by-step guide on how you can integrate it into your own React and Node.js application with minimal friction.
Why Clerk for Secure & Fast Authentication?
Before diving into the "how," let's cover the "why."
The Trouble with Rolling Your Own Auth
Building your own authentication system might seem tempting for ultimate control, but consider the hidden costs:
- Massive Time Investment: Developing, testing, and maintaining features like password resets, multi-factor authentication, and OAuth integrations takes significant development time.
- Security Expertise Required: Getting security right is hard. Vulnerabilities like improper password hashing, session hijacking risks, or insecure credential storage can have severe consequences.
- Ongoing Maintenance: Authentication libraries need constant updates to patch vulnerabilities. User management, compliance (like GDPR), and evolving security best practices add to the burden.
Unless authentication is your core product, building it yourself often diverts valuable resources from what truly differentiates your application.
Comparing Clerk to Alternatives
I evaluated several popular options before settling on Clerk:
Auth0
A powerful, enterprise-ready solution. However, for my relatively simple dashboard project, its extensive features and pricing felt like overkill, adding complexity I didn't need.
Firebase Auth
Excellent integration within the Google Cloud/Firebase ecosystem. But since my project relied on a PostgreSQL database (Neon) and a standard Node.js backend, tying auth tightly to Firebase felt less natural than a more agnostic solution.
Supabase Auth
Another great option, especially if you're already using the Supabase platform. Like Firebase, it's deeply integrated into its own ecosystem, which wasn't the best fit for my chosen stack (React/Node.js/Neon).
NextAuth.js (Auth.js)
Highly flexible and popular, especially within the Next.js community. It gives you fine-grained control but requires significantly more setup, configuration, and often building your own UI components compared to Clerk's out-of-the-box approach.
Key Clerk Advantages for This Project
Clerk hit the sweet spot for several reasons:
- Complete UI Components: Pre-built, customizable React components for Sign In, Sign Up, User Profile management (
<SignInButton>
,<UserButton>
, etc.) saved hours of frontend work.- Developer Experience (DX): Excellent TypeScript support, clear documentation, and intuitive React hooks (
useUser
,useAuth
) made integration incredibly smooth.- Speed of Implementation: Getting basic, secure authentication working took less than an hour, including the frontend UI.
- Security Focus: Offloading sensitive credential storage and complex security logic to a specialized service provided peace of mind.
- Backend Agnosticism: While offering framework-specific helpers, Clerk works well with various backends (like Node.js) through standard JWT verification and middleware.
Step-by-Step Clerk Integration Guide (React & Node.js Example)
Let's get practical. Here's how you can set up Clerk in a typical React frontend / Node.js backend application.
Prerequisites
- Clerk Account: Sign up for a free account at Clerk.dev.
- Create Application: Create a new application in your Clerk dashboard.
- API Keys: Find your Publishable Key (for the frontend) and Secret Key (for the backend) in the Clerk dashboard under "API Keys". [Placeholder: Screenshot of Clerk Dashboard API Keys section]
- Project Setup: Have a basic React project (e.g., created with Vite or Create React App) and a Node.js backend (e.g., using Express) ready.
Installation & Environment Variables
Install the necessary Clerk packages:
# In your React frontend project directory
npm install @clerk/clerk-react
# In your Node.js backend project directory
npm install @clerk/clerk-sdk-node
Create a .env.local file (or similar) in your frontend project root and add your Publishable Key:
# Frontend .env.local
VITE_CLERK_PUBLISHABLE_KEY=pk_test_YOUR_PUBLISHABLE_KEY
# Or for Create React App / Next.js:
# REACT_APP_CLERK_PUBLISHABLE_KEY=pk_test_YOUR_PUBLISHABLE_KEY
# NEXT_PUBLIC_CLERK_PUBLISHABLE_KEY=pk_test_YOUR_PUBLISHABLE_KEY
Create a .env file in your backend project root and add your Secret Key:
# Backend .env
CLERK_SECRET_KEY=sk_test_YOUR_SECRET_KEY
(Remember to add .env and .env.local to your .gitignore file!)
Frontend Setup: ClerkProvider & UI Components
In your main React application file (e.g., src/main.tsx or src/index.js), wrap your entire app with ClerkProvider:
// src/main.tsx (Example for Vite)
import React from "react";
import ReactDOM from "react-dom/client";
import App from "./App";
import { ClerkProvider } from "@clerk/clerk-react";
import "./index.css";
// Import your publishable key from env variables
const PUBLISHABLE_KEY = import.meta.env.VITE_CLERK_PUBLISHABLE_KEY;
if (!PUBLISHABLE_KEY) {
throw new Error("Missing Publishable Key");
}
ReactDOM.createRoot(document.getElementById("root")!).render(
<React.StrictMode>
<ClerkProvider publishableKey={PUBLISHABLE_KEY}>
<App />
</ClerkProvider>
</React.StrictMode>
);
Now, you can easily add login, logout, and user profile buttons anywhere in your app:
// Example component (e.g., Header.tsx)
import {
SignInButton,
SignUpButton,
UserButton,
SignedIn,
SignedOut,
} from "@clerk/clerk-react";
function Header() {
return (
<header
style={{
display: "flex",
justifyContent: "space-between",
padding: "1rem",
}}
>
<h1>My App</h1>
<div>
<SignedOut>
<SignInButton mode="modal" /> {/* Opens login in a modal */}
<SignUpButton mode="modal" /> {/* Opens sign up in a modal */}
</SignedOut>
<SignedIn>
<UserButton afterSignOutUrl="/" />{" "}
{/* Shows user avatar, menu, sign out */}
</SignedIn>
</div>
</header>
);
}
export default Header;
[Placeholder: Screenshot of Sign In / UserButton UI]
Use <SignedIn>
and <SignedOut>
to conditionally render content based on authentication status.
Protecting Routes with Clerk Middleware (Node.js Example)
On your Node.js backend (using Express as an example), you can protect specific API routes using Clerk's middleware:
// server.js (Node.js/Express Example)
require("dotenv").config();
const express = require("express");
const { ClerkExpressRequireAuth } = require("@clerk/clerk-sdk-node");
const app = express();
const port = process.env.PORT || 3001;
// Middleware to protect routes - requests without valid session/token will be rejected
// This should come BEFORE the routes you want to protect
app.use("/api/protected", ClerkExpressRequireAuth());
// Example of a protected route
app.get("/api/protected/data", (req, res) => {
// Thanks to the middleware, req.auth is populated
const userId = req.auth.userId;
console.log("Accessing protected data for user:", userId);
// Fetch data specific to the authenticated user
res.json({ message: `Hello user ${userId}, here is your protected data!` });
});
// Example of a public route
app.get("/api/public/info", (req, res) => {
res.json({ message: "This data is public." });
});
app.listen(port, () => {
console.log(`Server listening on port ${port}`);
});
(Note: Refer to the official [Link to Clerk Docs: Node SDK]Clerk Node SDK documentation[/Link] for the most up-to-date middleware implementation details and options.)
Accessing User Data on the Backend
As seen above, once a route is protected by Clerk middleware, the req.auth
object becomes available within your route handler. You can access details like:
req.auth.userId
: The unique ID for the authenticated user.req.auth.getToken()
: A function to retrieve the session token for downstream API calls.req.auth.claims
: Contains JWT claims, potentially including custom metadata.
Use req.auth.userId
to fetch user-specific data from your database (like Neon PostgreSQL in my case).
Real-World Experience: Pros & Cons
My experience integrating Clerk was overwhelmingly positive, but here's a balanced look:
The Good Surprises
- Rapid Setup: Seriously, basic auth UI and functionality were running in under 30 minutes.
- Excellent DX: The React hooks and TypeScript support made frontend integration seamless and type-safe.
- Helpful Dashboard: The Clerk developer dashboard is great for managing users, customizing settings, and testing different login flows during development.
Minor Hiccups & Workarounds
- UI Customization: While the pre-built components are convenient, extensive visual customization sometimes requires overriding default styles, which can be a bit fiddly. [Link to Clerk Docs: Theming/Components]
- SSR Complexity: Integrating Clerk with heavily Server-Side Rendered applications (like classic Next.js Pages Router) requires careful handling of authentication state on the server, often involving Clerk's server-side helpers. [Link to Clerk Docs: SSR/Next.js]
- Evolving Platform: As Clerk is newer than some competitors, very niche or advanced enterprise use cases might occasionally require custom solutions or workarounds, though their core offering is robust.
For my project's needs (dashboard behind a login, straightforward permissions), these were minor points.
The Payoff: Focusing on Your Core App
By using Clerk, I effectively outsourced the complexity of authentication. This allowed me to focus my limited development time entirely on building the core features of the SEO automation tool.
The benefits were clear:
- Secure user accounts and session management without manual setup.
- Pre-built, professional-looking login/signup/profile UI out of the box.
- Easy integration with social logins (Google, GitHub, etc.).
- No need to worry about password hashing, email verification flows, or JWT management.
- Scalable solution ready for more users or features later.
It felt like getting a major feature for free, letting me ship the core value proposition much faster.
Is Clerk Right For You?
Clerk shines brightest for:
- Solo Developers & Indie Hackers: Massively reduces the burden of implementing secure auth.
- Startups & MVPs: Allows teams to focus on core features and validate ideas quickly.
- Projects Needing Standard Auth Flows: Ideal for apps requiring typical login, signup, profile management, and route protection without deeply complex custom requirements.
- React/Next.js Ecosystem: Has particularly strong support and tooling for these frameworks.
If you need extremely granular control over every aspect of the auth UI and flow, or have very complex, unique enterprise requirements, alternatives like Auth.js (NextAuth.js) or Auth0 might offer more flexibility, albeit with increased setup time.
Conclusion & Next Steps
Migrating authentication away from a custom build (or avoiding one altogether) using a service like Clerk can be a huge productivity booster. It provides robust security and a polished user experience with minimal development effort.
For the SEO automation app, Clerk proved to be the perfect fit, handling user management securely while I focused on the data processing and dashboard features.
In the next post, I'll dive into how I tackled the core challenge: transforming those messy Excel sheets into a clean, interactive React dashboard using the data fetched via our Node.js backend. Stay tuned!
Related Tags: Clerk, Authentication - React - Node.js - Web App Security - Tutorial - Setup Guide - Indie Dev - Auth Comparison - JWT